Login with Google Account in PHP
- Tech Area
- February 6, 2024
Nowadays Google login is very common on website and easy to use. Most of the website users do not want to fill long registration forms details. So most of the developers provide a Google login button on their websites.
If the user has a Google account and don’t want to fill long details then they can log in to the website with own Google account. Google provides client library for PHP to access Google APIs. We can easily integrate to the website.
File Structure with Google PHP SDK Class
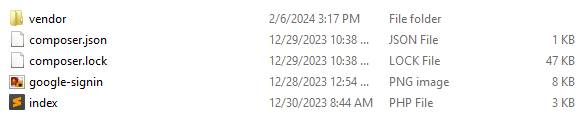
Installation Google APIs Client Library for PHP
Google APIs client library can be installed with Composer. Run this command in your project root to install this library:
composer require google/apiclient:^2.15.0
Below are the step by step process of how to create Client ID and Client secret.
Step 1: Create Google API Console Project
To create Google API console project simply go to http://console.developers.google.com/ and click on CREATE PROJECT.
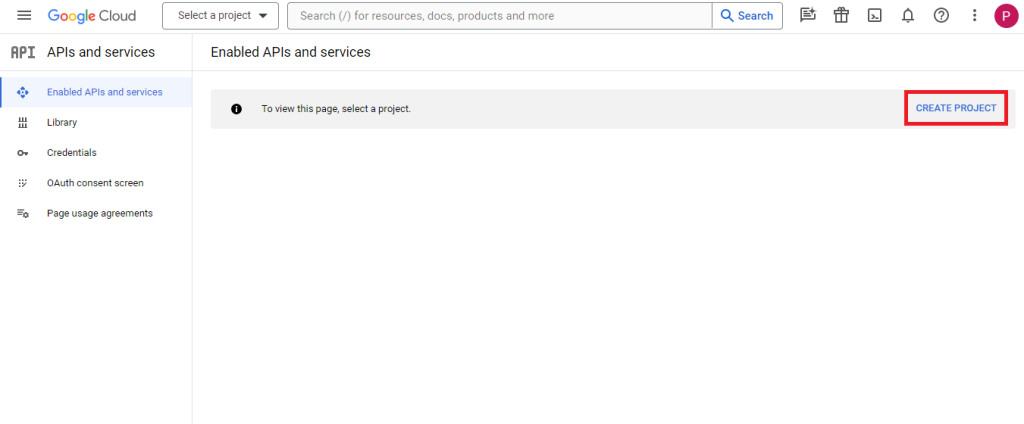
Enter the Project name then click on CREATE button.
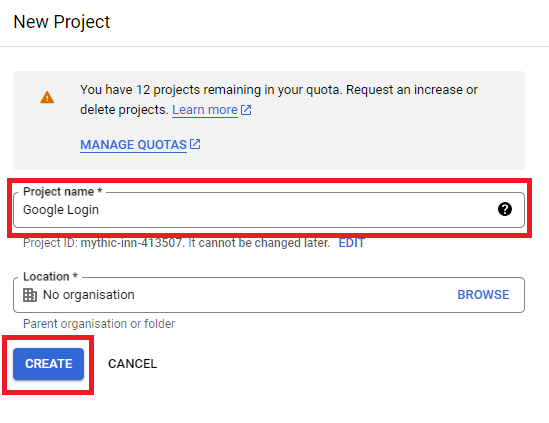
Now go to OAuth consent screen choose User Type as External then click on CREATE button.
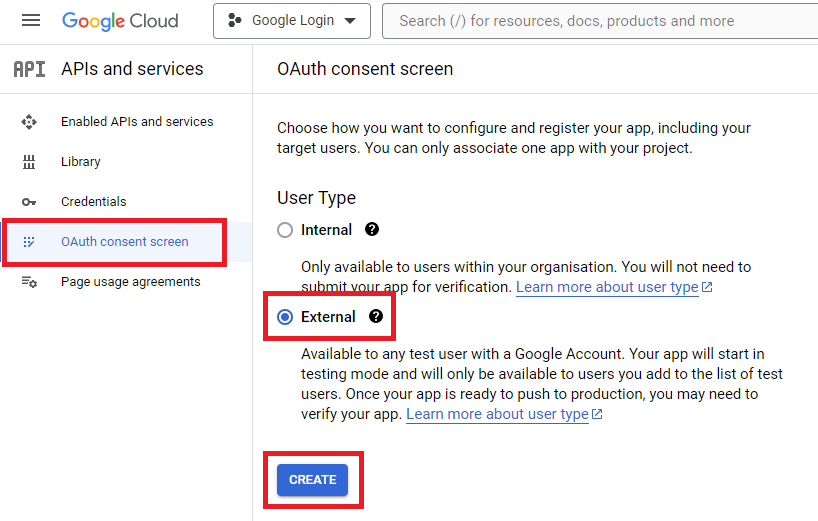
Now enter mandatory fields App name, User support email, Email addresses in Developer contact information then click on SAVE AND CONTINUE button.
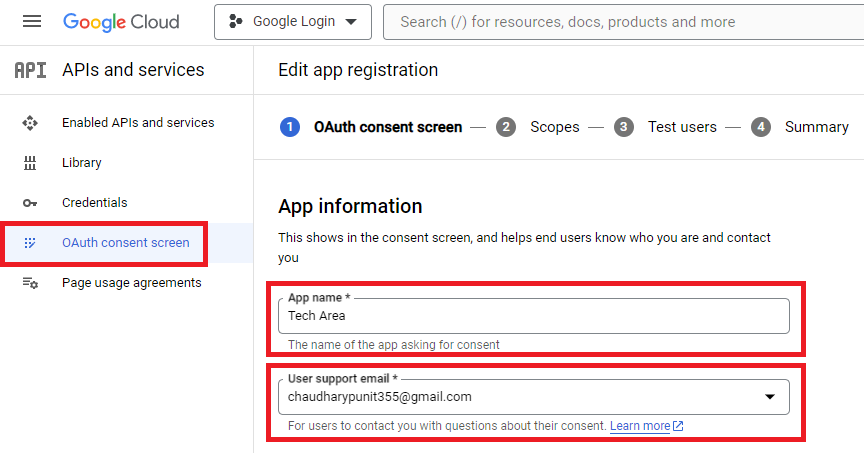
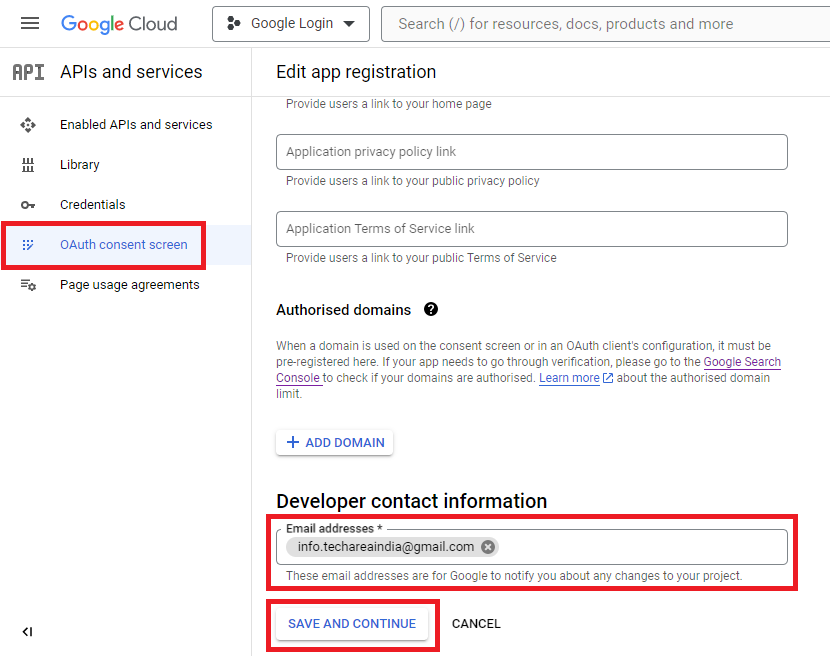
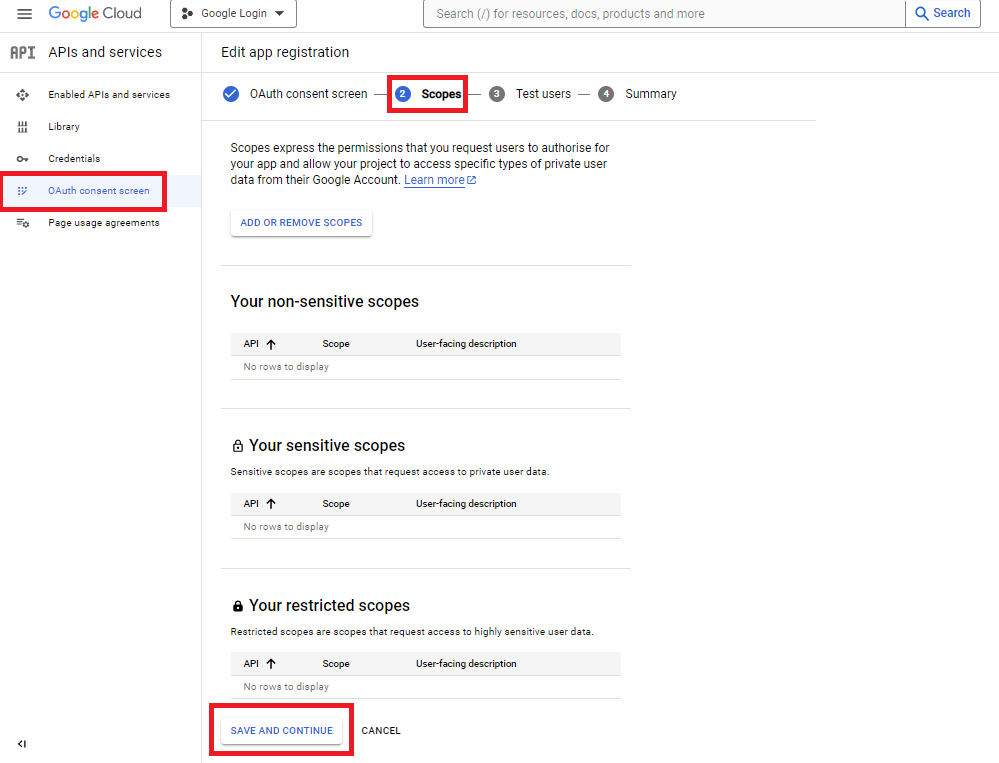
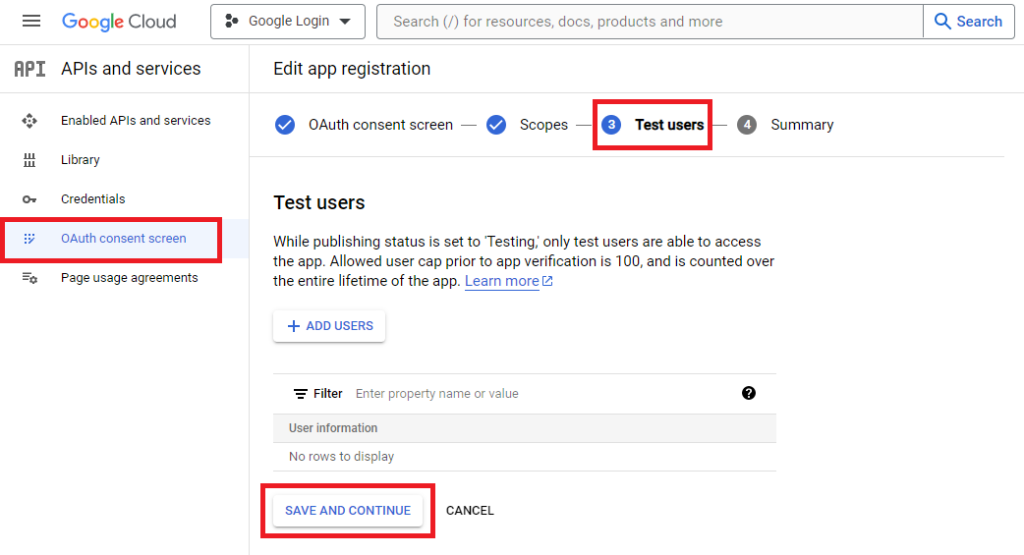
Then click on BACK TO DASHBOARD
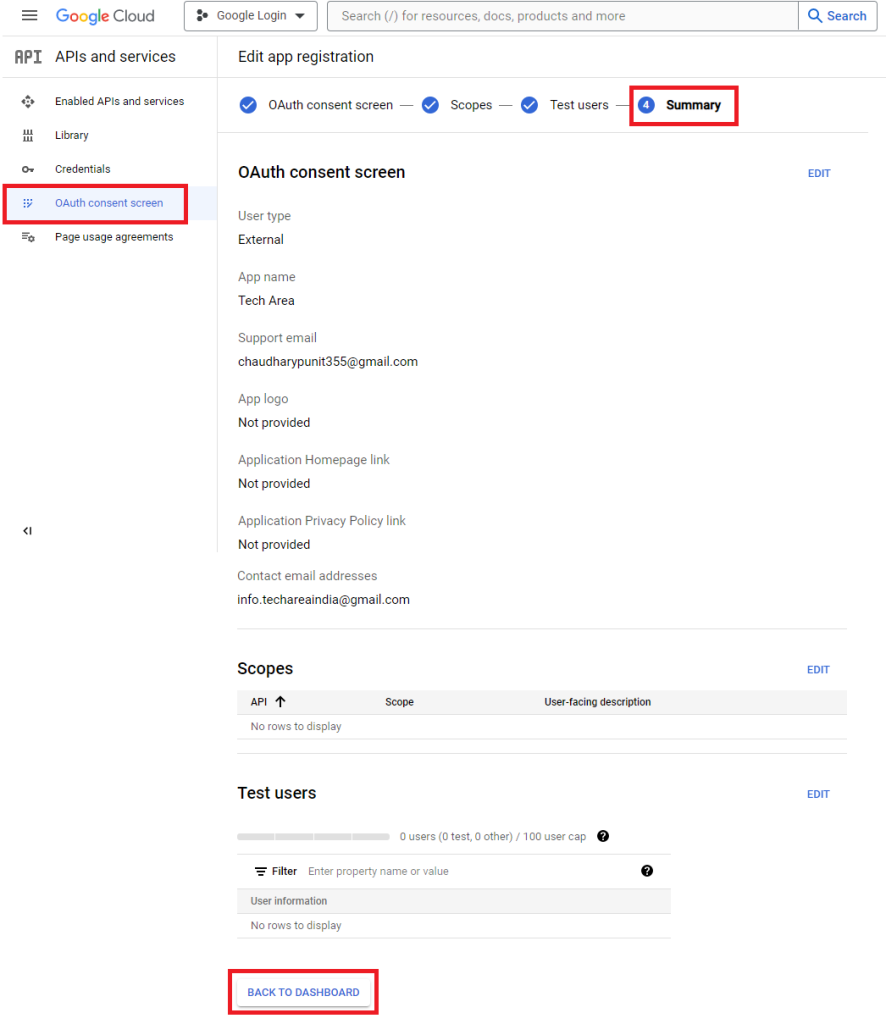
Now click on Credentials button.
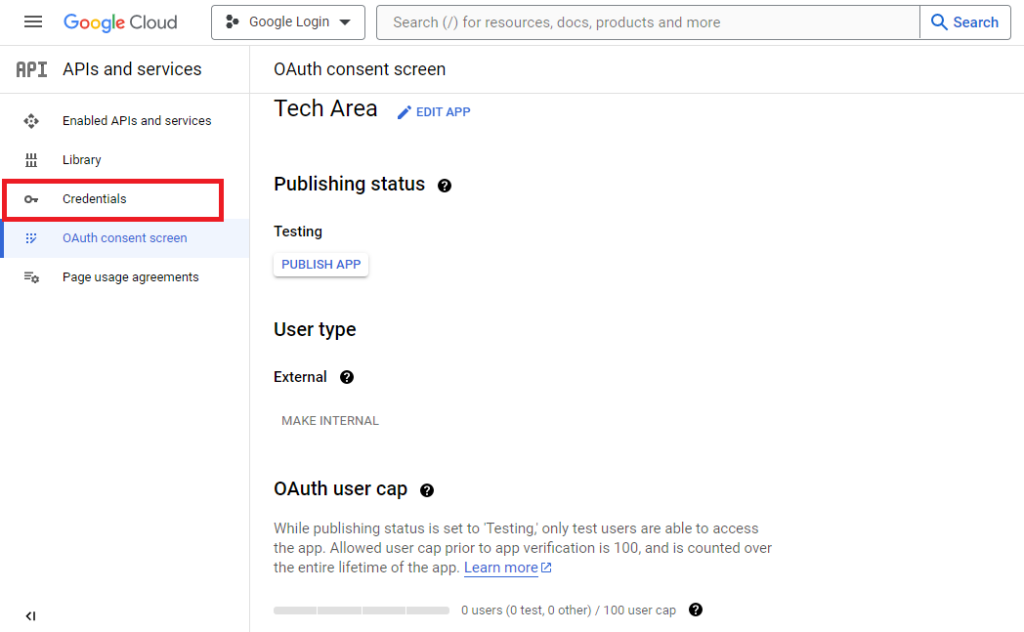
Click on CREATE CREDENTIALS then click on OAUTH client ID.
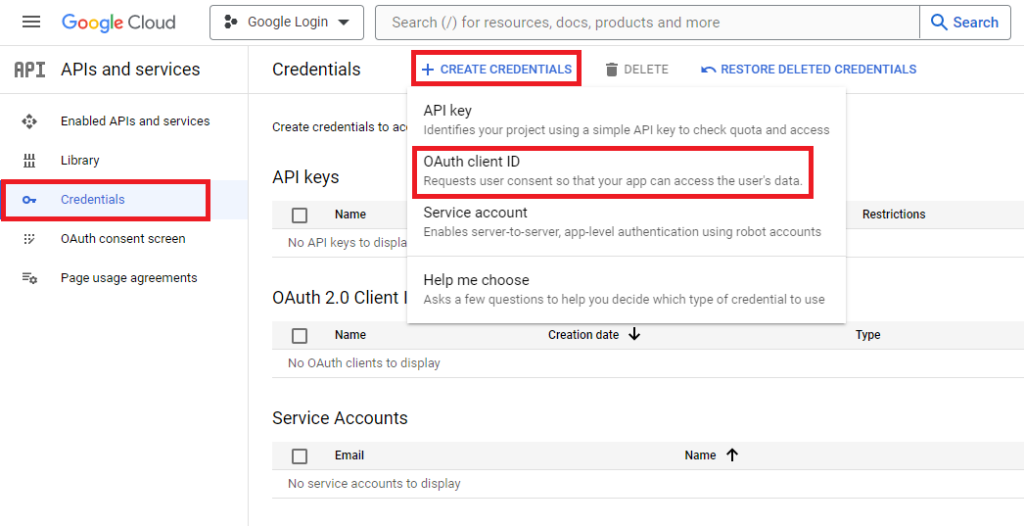
Choose Application type as Web application and enter the Name, Authorised redirect URIs then click on CREATE button.
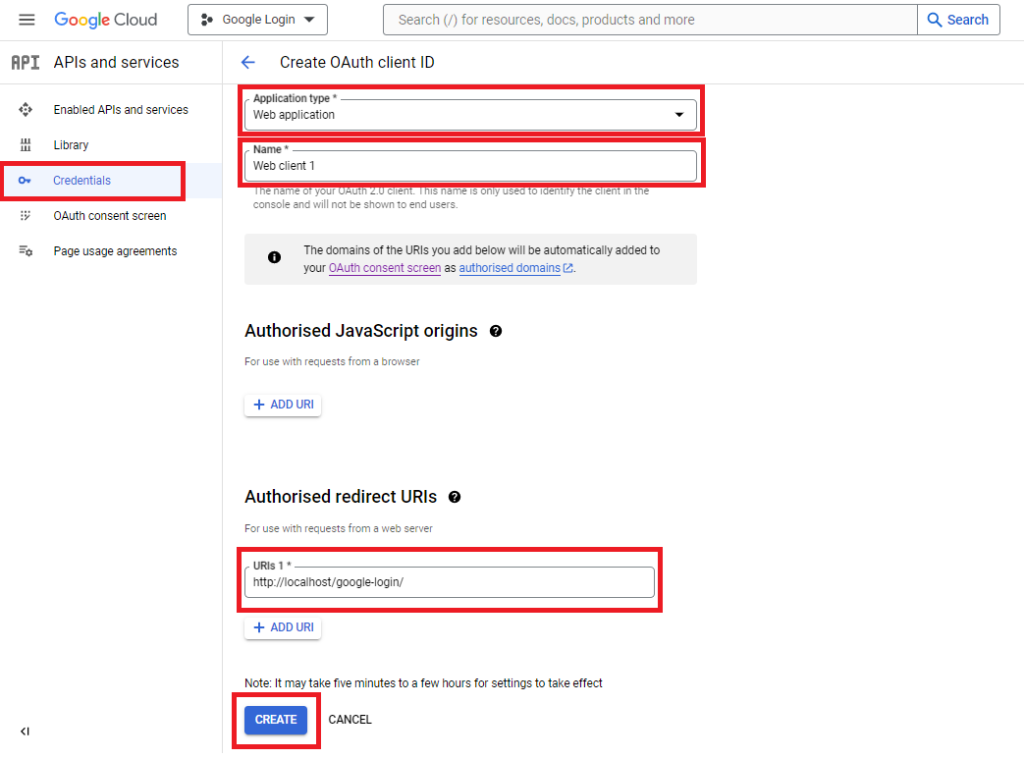
A dialog box will appear with OAuth client details, note the Client ID and Client secret. This Client ID and Client secret allow you to access the Google APIs.
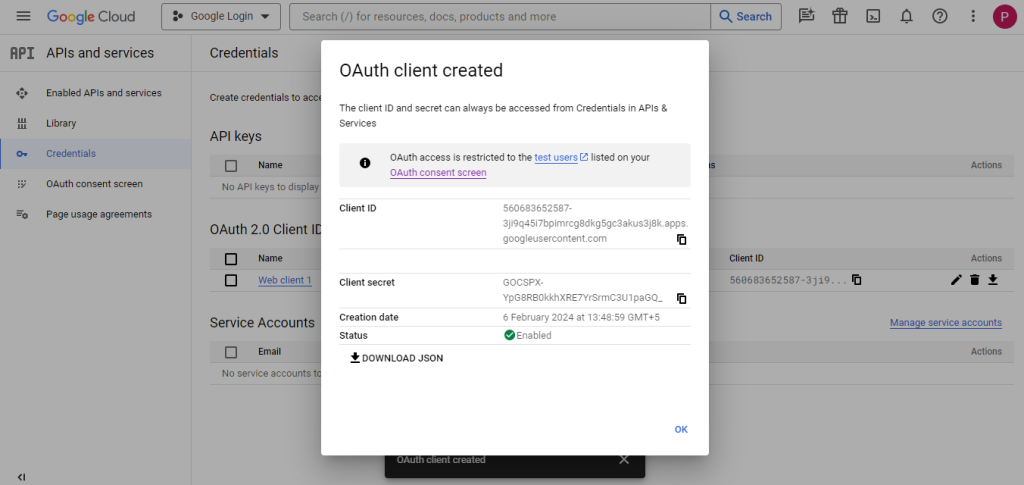
Note that: This Client ID and Client secret need to be specified in the script at the time of Google API call. Also, the Authorized redirect URI needs to be matched with the redirect URL that specified in the script.
Step 2: Create index.php file
<html>
<head>
<title>Login Form</title>
<link rel="stylesheet" href="https://maxcdn.bootstrapcdn.com/bootstrap/3.3.6/css/bootstrap.min.css" />
</head>
<style>
.box
{
width:100%;
max-width:400px;
background-color:#f9f9f9;
border:1px solid #ccc;
border-radius:5px;
padding:16px;
margin:0 auto;
}
</style>
<body>
<?php
require_once 'vendor/autoload.php';
// init configuration
$clientID = ''; // your client id
$clientSecret = ''; // your client secret
$redirectUri = 'http://localhost/google-login/';
// create Client Request to access Google API
$client = new Google_Client();
$client->setClientId($clientID);
$client->setClientSecret($clientSecret);
$client->setRedirectUri($redirectUri);
$client->addScope("email");
$client->addScope("profile");
// authenticate code from Google OAuth Flow
if (isset($_GET['code'])) {
$token = $client->fetchAccessTokenWithAuthCode($_GET['code']);
$client->setAccessToken($token['access_token']);
// get profile info
$google_oauth = new Google_Service_Oauth2($client);
$google_account_info = $google_oauth->userinfo->get();
$email = $google_account_info->email;
$name = $google_account_info->name;
?>
<div class="container">
<div class="box">
<div class="form-group">
<label for="email">Emailid: <?php echo $email; ?></label>
<label for="name">Name: <?php echo $name; ?></label>
</div>
</div>
</div>
<?php } else {?>
<div class="container">
<div class="table-responsive">
<h3 align="center">Login using Google with PHP</h3>
<div class="box">
<div class="form-group">
<label for="email">Emailid</label>
<input type="text" name="email" id="email" placeholder="Enter Email" class="form-control" required />
</div>
<div class="form-group">
<label for="password">Password</label>
<input type="password" name="pwd" id="pwd" placeholder="Enter Password" class="form-control"/>
</div>
<div class="form-group">
<input type="submit" id="login" name="login" value="Login" class="btn btn-success form-control"/>
<hr>
<center><a href="<?php echo $client->createAuthUrl() ?>"><img src="google-signin.png" width="256"></a></center>
</div>
</div>
</div>
</div>
<?php } ?>
</body>
</html>
Publish Google API Console Project
Once the Google login integration is completed and the authentication process is working properly, you need to make the Google API Console Project public.
-While the publishing status is set to Testing, only specified test users are able to access this app.
-While the publishing status is set to Public, this app is available for all Google users.
You need to submit the application for verification to make the Google Cloud Console project public.
Navigate to the OAuth consent screen and click the PUBLISH APP button.
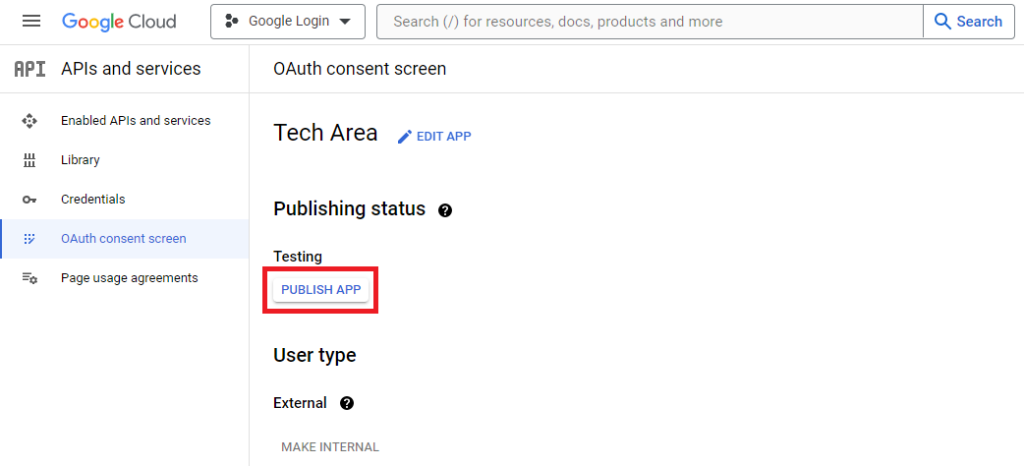
Download Source Code
Join 10,000+ subscriber