Dropdown value insert, edit & update in PHP & MySQL
- Tech Area
- February 7, 2024
In this tutorials, We will learn how to insert dropdown value and update it in PHP and MySQL Database.
Files used in this tutorial:
1- connection.php (database connection file)
2- index.php (registration form with dropdown)
3- show-details.php (fetch and show data)
4- edit-details.php (update data)
Below are the step by step process of how to insert and update dropdown value in PHP and MySQL.
Step 1: Create a Database connection
In this step, create a new file connection.php to create database connection.
connection.php
<?php
$server = "localhost";
$username = "root";
$password = "";
$database = "college_db";
$connection = mysqli_connect("$server","$username","$password");
$select_db = mysqli_select_db($connection, $database);
if(!$select_db)
{
echo("connection terminated");
}
?>
Step 2: Create Registration form
In this step, create a new file index.php. This is the main file used to create registration form and insert value into database.
This screenshot shows the registration form with dropdown.
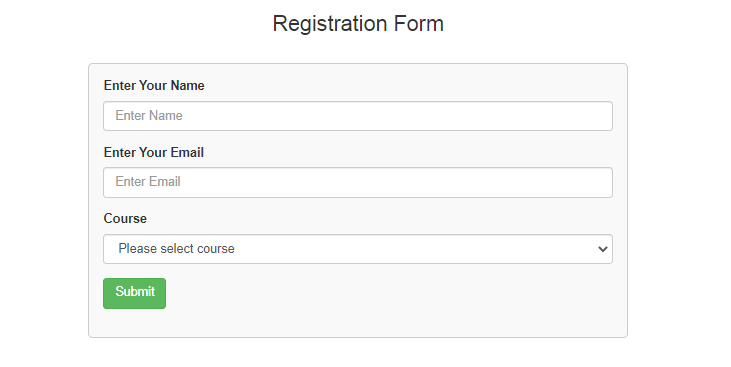
index.php
<html>
<head>
<title>Registration Form</title>
<link rel="stylesheet" href="https://maxcdn.bootstrapcdn.com/bootstrap/3.3.6/css/bootstrap.min.css" />
</head>
<style>
.box
{
width:100%;
max-width:600px;
background-color:#f9f9f9;
border:1px solid #ccc;
border-radius:5px;
padding:16px;
margin:0 auto;
}
.error
{
color: red;
font-weight: 700;
}
</style>
<?php
include('connection.php');
if(isset($_REQUEST['register']))
{
$name = $_POST['name'];
$email = $_POST['email'];
$course = $_POST['course'];
$insert_query = mysqli_query($connection,"insert into tbl_registration set name='$name', emailid='$email', course='$course'");
if($insert_query>0)
{
$msg = "Data inserted successfully";
}
else
{
$msg = "Error!";
}
}
?>
<body>
<div class="container">
<div class="table-responsive">
<h3 align="center">Registration Form</h3>
<a href="show-details.php">View Record</a>
<div class="box">
<form method="post">
<div class="form-group">
<label for="name">Enter Your Name</label>
<input type="text" name="name" id="name" placeholder="Enter Name" required class="form-control"/>
</div>
<div class="form-group">
<label for="email">Enter Your Email</label>
<input type="email" name="email" id="email" placeholder="Enter Email" required class="form-control"/>
</div>
<div class="form-group">
<label for="course">Course</label>
<select name="course" required class="form-control">
<option value="">Please select course</option>
<?php
$select_query = mysqli_query($connection,"select name from tbl_course");
while($result = mysqli_fetch_array($select_query)){?>
<option><?php echo $result['name']; ?></option>
<?php } ?>
</select>
</div>
<div class="form-group">
<input type="submit" id="register" name="register" value="Submit" class="btn btn-success" />
</div>
<p class="error"><?php if(!empty($msg)){ echo $msg; } ?></p>
</form>
</div>
</div>
</div>
</body>
</html>
Step 3: Fetch and Show data
In this step, create a new file show-details.php. This file is used to fetch and show data from the database.
show-details.php
<style>
table {
font-family: arial, sans-serif;
border-collapse: collapse;
width: 50%;
}
td, th {
border: 1px solid #dddddd;
text-align: left;
padding: 8px;
}
tr:nth-child(even) {
background-color: #dddddd;
}
</style>
<table>
<tr>
<th>Name</th>
<th>EmailId</th>
<th>Course</th>
<th>Action</th>
</tr>
<?php
include('connection.php');
$select_query = mysqli_query($connection,"select * from tbl_registration");
while($result = mysqli_fetch_array($select_query)){
?>
<tr>
<td><?php echo $result['name']; ?></td>
<td><?php echo $result['emailid']; ?></td>
<td><?php echo $result['course']; ?></td>
<td><a href="edit-details.php?ids=<?php echo $result['id'];?>">Edit</a></td>
</tr>
<?php } ?>
</table>
Step 4: Update form data
In this step, create a new file edit-details.php. This file is used to update form data.
edit-details.php
<html>
<head>
<title>Registration Form</title>
<link rel="stylesheet" href="https://maxcdn.bootstrapcdn.com/bootstrap/3.3.6/css/bootstrap.min.css" />
</head>
<style>
.box
{
width:100%;
max-width:600px;
background-color:#f9f9f9;
border:1px solid #ccc;
border-radius:5px;
padding:16px;
margin:0 auto;
}
.error
{
color: red;
font-weight: 700;
}
</style>
<body>
<div class="container">
<div class="table-responsive">
<h3 align="center">Registration Form</h3><br/>
<a href="show-details.php">View Details</a>
<div class="box">
<?php
include('connection.php');
$ids = $_GET['ids'];
if(isset($_REQUEST['update']))
{
$name = $_POST['name'];
$email = $_POST['email'];
$course = $_POST['course'];
$update_query = mysqli_query($connection,"update tbl_registration set name='$name', emailid='$email', course='$course' where id='$ids'");
if($update_query>0)
{
$msg = "Data updated successfully";
}
else
{
$msg = "Error!";
}
}
$select_query = mysqli_query($connection,"select * from tbl_registration where id='$ids'");
$res = mysqli_fetch_array($select_query);
?>
<form method="post" >
<div class="form-group">
<label for="name">Enter Your Name</label>
<input type="text" name="name" id="name" placeholder="Enter Name" required class="form-control" value="<?php echo $res['name']; ?>"/>
</div>
<div class="form-group">
<label for="email">Enter Your Email</label>
<input type="email" name="email" id="email" placeholder="Enter Email" required class="form-control" value="<?php echo $res['emailid']; ?>"/>
</div>
<div class="form-group">
<label for="course">Course</label>
<select name="course" required class="form-control">
<option value="">Please select course</option>
<?php
$select_query = mysqli_query($connection,"select name from tbl_course");
while($result = mysqli_fetch_array($select_query)){?>
<option <?php if($result['name']==$res['course']){?> selected="selected"; <?php } ?>><?php echo $result['name']; ?></option>
<?php } ?>
</select>
</div>
<div class="form-group">
<input type="submit" id="update" name="update" value="Update" class="btn btn-success"/>
</div>
<p class="error"><?php if(!empty($msg)){ echo $msg; } ?></p>
</form>
</div>
</div>
</div>
</body>
</html>
Download Source Code
Join 10,000+ subscriber