CRUD operation with multiple images using AJAX in PHP
- Tech Area
- June 21, 2024
In this post, We will see how to perform create, read, update and delete operations with multiple images using AJAX in PHP. CRUD operations are the most used functionality in the web application managed dynamically. The add, edit, and delete functionality helps to manage data with the database on the website. If you are working with images, CRUD functionality can be used to manage the images.
Files used in this tutorial:
1- connection.php (database connection file)
2- index.php (HTML table)
3- insert-data.php (data submit into database)
4- update-data.php (update data)
5- delete-data.php (delete image)
6- delete-product.php (delete data from database)
7- show-data.php (fetch all data from the database)
8- get-data.php (fetch single data)
Below are the step by step process of how to perform CRUD operation with multiple images using AJAX in PHP and MySQL.
Step 1: Create a Database connection
In this step, create a new file connection.php to create database connection.
connection.php
<?php
$server = "localhost";
$username = "root";
$password = "";
$database = "college_db";
$connection = mysqli_connect("$server","$username","$password");
$select_db = mysqli_select_db($connection, $database);
if(!$select_db)
{
echo("connection terminated");
}
?>
Step 2: Create a file for show data in HTML Table
Now create a new file index.php This is the main file used for show data in HTML table.
This screenshot shows the HTML table.
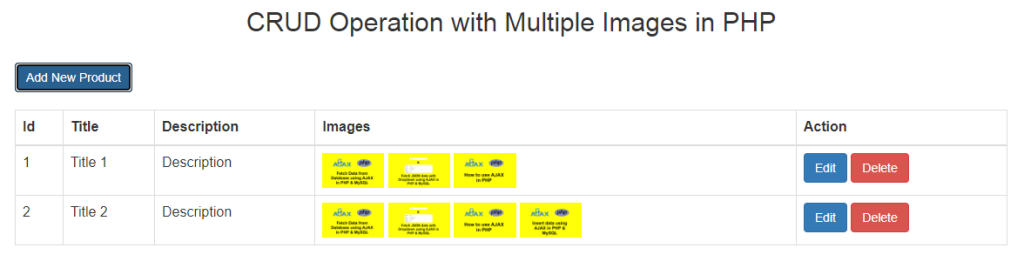
index.php
First of all include necessary CSS and JavaScript library.
<link rel="stylesheet" type="text/css" href="style.css">
<link rel="stylesheet" href="https://maxcdn.bootstrapcdn.com/bootstrap/3.3.6/css/bootstrap.min.css"/>
<script src="https://code.jquery.com/jquery-3.7.1.js"></script>
<script src="https://cdn.jsdelivr.net/npm/bootstrap@3.3.7/dist/js/bootstrap.min.js"></script>
Now create HTML table.
<div class="container">
<h2 class="text-center">CRUD Operation with Multiple Images in PHP</h2>
<br/>
<button type="button" class="btn btn-primary" data-toggle="modal" data-target="#addProduct">Add New Product</button>
<br/><br/>
<table class="table table-bordered">
<thead>
<th>Id</th>
<th>Title</th>
<th>Description</th>
<th>Images</th>
<th>Action</th>
</thead>
<tbody id="result">
</tbody>
</table>
</div>
Now use jquery AJAX script.
<script type="text/javascript">
$(document).ready(function(){
showdata();
getdata();
$("#addProductdetail").on("click", function(e){
e.preventDefault();
var formData = new FormData($('#uploadimage')[0]);
$.ajax({
url: 'insert-data.php',
method: 'post',
data: formData,
contentType: false,
processData: false,
success: function(data){
$("#msg").html(data);
$("form").trigger('reset');
showdata();
}
});
});
});
function showdata(){
$.ajax({
url: 'show-data.php',
method: 'post',
success: function(result)
{
$("#result").html(result);
}
});
}
function getdata(){
$(document).on("click","#edit_product",function(){
var id = $(this).attr('data-id');
$.ajax({
url: 'get-data.php',
method: 'post',
data: {userid: id},
dataType: 'JSON',
success: function(data)
{
$('#editproduct').modal('show');
$('#edit_title').val(data.title);
$('#edit_desc').val(data.description);
$('#productId').val(data.id);
$('#edit_image').empty();
var imageList = data.image.split(',').map(function(item) {
return item.trim();
});
for (var i = 0; i < imageList.length; i++) {
var imageUrl = 'uploads/' + imageList[i];
var imageContainer = $('<div class="image-container">');
var imageElement = $('<img class="image-item" src="' + imageUrl + '">');
var deleteButton = $('<button id="dlt-btn" class="btn btn-danger btn-xs dlt_btn" data-id="'+ id +'" data-image="' + imageList[i] + '">Delete</button>');
imageContainer.append(imageElement);
imageContainer.append(deleteButton);
$('#edit_image').append(imageContainer);
}
}
});
});
}
$(document).ready(function(){
function deleteImage(image,id,imageContainer) {
$.ajax({
url: 'delete-data.php',
method: 'post',
data: {image_url: image, id: id},
success: function(data)
{
imageContainer.remove();
}
});
}
$(document).on("click","#dlt-btn",function(e){
e.preventDefault();
var image = $(this).data('image');
var id = $(this).data('id');
var imageContainer = $(this).closest('.image-container');
if (confirm('Are you sure you want to delete this image?')) {
deleteImage(image,id,imageContainer);
}
});
});
$(document).ready(function(){
function deleteProduct(id) {
$.ajax({
url: 'delete-product.php',
method: 'post',
data: {id: id},
success: function(data)
{
alert(data);
showdata();
}
});
}
$(document).on("click","#deleteproduct",function(e){
var id = $(this).data('id');
if (confirm('Are you sure you want to delete this Product?')) {
deleteProduct(id);
}
});
});
$(document).ready(function(){
$("#updateProduct").on("click", function(e){
e.preventDefault();
var formData = new FormData($('#editimage')[0]);
$.ajax({
url: 'update-data.php',
method: 'post',
data: formData,
contentType: false,
processData: false,
success: function(data){
$("#editmsg").html(data);
showdata();
}
});
});
});
$(document).on("click","#close_btn",function(e){
$('#msg').html('');
$('#editmsg').html('');
showdata();
});
</script>
Now create modal for add and edit product.
<!-- Add Product Modal -->
<div class="modal fade" id="addProduct" tabindex="-1" role="dialog" aria-labelledby="exampleModalLabel" aria-hidden="true">
<div class="modal-dialog" role="document">
<div class="modal-content">
<div class="modal-body">
<div class="box">
<form id="uploadimage" enctype="multipart/form-data">
<div class="form-group">
<label for="title">Title</label>
<input type="text" name="title" id="title" placeholder="Enter Title" required class="form-control"/>
</div>
<div class="form-group">
<label for="description">Description</label>
<textarea name="description" id="description" placeholder="Enter Description" required class="form-control"></textarea>
</div>
<div class="form-group">
<label for="image">Select Images</label>
<input type="file" name="images[]" id="images" class="form-control" multiple/>
</div>
<div class="form-group">
<input type="submit" id="addProductdetail" name="addProduct" value="Submit" class="btn btn-success"/>
<button type="button" class="btn btn-danger" data-dismiss="modal" id="close_btn">Close</button>
</div>
<p class="msg" id="msg"></p>
</form>
</div>
</div>
</div>
</div>
</div>
<!--Edit Product Modal -->
<div class="modal fade" id="editproduct" tabindex="-1" role="dialog" aria-labelledby="exampleModalLabel" aria-hidden="true">
<div class="modal-dialog" role="document">
<div class="modal-content">
<div class="modal-body">
<div class="box">
<form id="editimage" enctype="multipart/form-data">
<div class="form-group">
<label for="title">Title</label>
<input type="text" name="title" id="edit_title" placeholder="Enter Title" required class="form-control"/>
</div>
<div class="form-group">
<label for="description">Description</label>
<textarea name="description" id="edit_desc" placeholder="Enter Description" required class="form-control"></textarea>
</div>
<div class="form-group">
<label for="image">Images</label>
<div id="edit_image" class="image-gallery"></div>
</div>
<div class="form-group">
<label for="image">Select Images</label>
<input type="file" name="images[]" id="images" class="form-control" multiple/>
</div>
<div class="form-group">
<input type="hidden" name="productId" id="productId">
<input type="submit" id="updateProduct" name="updateProduct" value="Update" class="btn btn-success" />
<button type="button" class="btn btn-danger" data-dismiss="modal" id="close_btn">Close</button>
</div>
<p class="editmsg" id="editmsg"></p>
</form>
</div>
</div>
</div>
</div>
</div>
Step 3: Create new file for insert data
In this step, create a new file insert-data.php. This file used to insert data into the database.
insert-data.php
<?php
include('connection.php');
if(isset($_FILES['images']))
{
$title = $_POST['title'];
$desc = $_POST['description'];
foreach($_FILES['images']['tmp_name'] as $key => $tmp_name)
{
$file_name = $_FILES['images']['name'][$key];
$file_tmp = $_FILES['images']['tmp_name'][$key];
$file_destination = 'uploads/'.$file_name;
move_uploaded_file($file_tmp, $file_destination);
}
$images = implode(', ',$_FILES['images']['name']);
$insert_image = mysqli_query($connection, "insert into tbl_product set title='$title', description='$desc', image='$images'");
echo "Product Added Successfully.";
}
?>
Step 4: Create new file for update data
In this step, create a new file update-data.php. This file used to update data into the database.
update-data.php
<?php
include('connection.php');
if(isset($_POST['productId'])){
$id = $_POST['productId'];
$title = $_POST['title'];
$desc = $_POST['description'];
$update_data = mysqli_query($connection, "update tbl_product set title='$title', description='$desc' where id='$id'");
if(isset($_FILES['images'])) {
$select_images = mysqli_query($connection, "select image from tbl_product where id='$id'");
$res = mysqli_num_rows($select_images);
if($res>0)
{
$row = mysqli_fetch_array($select_images);
$existing_image_str = $row['image'];
$existing_images = explode(', ',$existing_image_str);
foreach($_FILES['images']['tmp_name'] as $key => $tmp_name)
{
$file_name = $_FILES['images']['name'][$key];
$file_tmp = $_FILES['images']['tmp_name'][$key];
$file_destination = 'uploads/'.$file_name;
move_uploaded_file($file_tmp, $file_destination);
}
$existing_images[] = $file_name;
$images = implode(', ',$existing_images);
$update_data = mysqli_query($connection, "update tbl_product set image='$images' where id='$id'");
echo "Product Updated Successfully.";
}
}
}
?>
Step 5: Create new file for delete image
In this step, create a new file delete-data.php. This file used to delete image from the database and directory also.
delete-data.php
<?php
include("connection.php");
$image = $_POST['image_url'];
$id = $_POST['id'];
$select_query = mysqli_query($connection, "select image from tbl_product where id='$id'");
$row = mysqli_num_rows($select_query);
if($row>0)
{
$res = mysqli_fetch_assoc($select_query);
$image_list = explode(', ',$res['image']);
$key = array_search($image, $image_list);
if ($key !== false) {
unset($image_list[$key]);
}
$updated_image_column = implode(', ',$image_list);
$update_query = mysqli_query($connection, "UPDATE tbl_product SET image = '$updated_image_column' WHERE id='$id'");
$image_path = 'uploads/' . $image;
if (file_exists($image_path)) {
unlink($image_path);
}
}
?>
Step 6: Create new file for delete product
In this step, create a new file delete-product.php. This file used to delete product from the database.
delete-product.php
<?php
include("connection.php");
$id = $_POST['id'];
$delete_query = mysqli_query($connection, "delete from tbl_product where id='$id'");
if($delete_query>0)
{
echo "Product Deleted Successfully.";
}
?>
Step 7: Create new file for fetch all data
In this step, create a new file show-data.php. This file used to fetch all data from the database.
show-data.php
<?php
include("connection.php");
$fetch_query = mysqli_query($connection, "select * from tbl_product");
$row = mysqli_num_rows($fetch_query);
if($row>0)
{
while($res = mysqli_fetch_array($fetch_query))
{?>
<tr>
<td><?php echo $res['id']; ?></td>
<td><?php echo $res['title']; ?></td>
<td><?php echo $res['description']; ?></td>
<td>
<?php
$image = explode(', ',$res['image']);
foreach ($image as $images){?>
<img src="uploads/<?php echo $images; ?>" height="40">
<?php } ?>
</td>
<td><button type="button" class="btn btn-primary" id="edit_product" data-id="<?php echo $res['id']; ?>">Edit</button>
<button type="button" class="btn btn-danger" id="deleteproduct" data-id="<?php echo $res['id']; ?>">Delete</button></td>
</tr>
<?php }
}
?>
Step 8: Create new file for fetch single data
In this step, create a new file get-data.php. This file used to fetch single data from the database based on product id.
get-data.php
<?php
include("connection.php");
$id = $_POST['userid'];
$fetch_query = mysqli_query($connection, "select * from tbl_product where id='$id'");
$row = mysqli_num_rows($fetch_query);
if($row>0)
{
$res = mysqli_fetch_array($fetch_query);
echo json_encode($res);
}
?>
Download Source Code
Join 10,000+ subscriber