How to use jQuery DataTable in PHP and MySQL
- Tech Area
- April 5, 2024
In this post, We will discuss how to use jQuery DataTable in PHP and MySQL. If you want to show fetch data from database and show in table form on web page, then you can achieve this task using jQuery DataTable plugin.
Files used in this tutorial:
1- connection.php (database connection file)
2- index.php (fetch data from database)
Below are the step by step process of how to use jQuery DataTable in PHP and MySQL.
Step 1: Create a Database connection
In this step, create a new file connection.php to create database connection.
connection.php
<?php
$server = "localhost";
$username = "root";
$password = "";
$database = "college_db";
$connection = mysqli_connect("$server","$username","$password");
$select_db = mysqli_select_db($connection, $database);
if(!$select_db)
{
echo("connection terminated");
}
?>
Step 2: Create a file for fetch data and initialise DataTable
Now create a new file index.php This is the main file used for fetch data from database. First, We will create an HTML Table.
index.php
First of all include necessary CSS and JavaScript library.
<link rel="stylesheet" type="text/css" href="//cdn.datatables.net/2.0.3/css/dataTables.dataTables.min.css">
<script src="https://code.jquery.com/jquery-3.7.1.min.js"></script>
<script src="//cdn.datatables.net/2.0.3/js/dataTables.min.js"></script>
Now create body for Table.
<body>
<table id="myTable">
<thead>
<tr>
<th>Name</th>
<th>Course</th>
<th>Country</th>
</tr>
</thead>
<tbody>
<?php
include('connection.php');
$select_query = mysqli_query($connection,"select * from tbl_student");
while($data = mysqli_fetch_array($select_query)){
?>
<tr>
<td><?php echo $data['name']; ?></td>
<td><?php echo $data['course']; ?></td>
<td><?php echo $data['country']; ?></td>
</tr>
<?php } ?>
</tbody>
</table>
</body>
After this, create script for initialise datatable.
<script>
$(document).ready(function(){
$('#myTable').DataTable();
});
</script>
Source Code
Here is the full code that we have written for index.php.
<!DOCTYPE html>
<html>
<head>
<meta charset="utf-8">
<meta name="viewport" content="width=device-width, initial-scale=1">
<title>jQuery DataTables</title>
<style>
table {
font-family: arial, sans-serif;
border-collapse: collapse;
width: 100%;
}
td, th {
border: 1px solid #dddddd;
text-align: left;
padding: 8px;
}
tr:nth-child(even) {
background-color: #dddddd;
}
</style>
<link rel="stylesheet" type="text/css" href="//cdn.datatables.net/2.0.3/css/dataTables.dataTables.min.css">
</head>
<body>
<table id="myTable">
<thead>
<tr>
<th>Name</th>
<th>Course</th>
<th>Country</th>
</tr>
</thead>
<tbody>
<?php
include('connection.php');
$select_query = mysqli_query($connection,"select * from tbl_student");
while($data = mysqli_fetch_array($select_query)){
?>
<tr>
<td><?php echo $data['name']; ?></td>
<td><?php echo $data['course']; ?></td>
<td><?php echo $data['country']; ?></td>
</tr>
<?php } ?>
</tbody>
</table>
<script src="https://code.jquery.com/jquery-3.7.1.min.js"></script>
<script src="//cdn.datatables.net/2.0.3/js/dataTables.min.js"></script>
<script>
$(document).ready(function(){
$('#myTable').DataTable();
});
</script>
</body>
</html>
Output
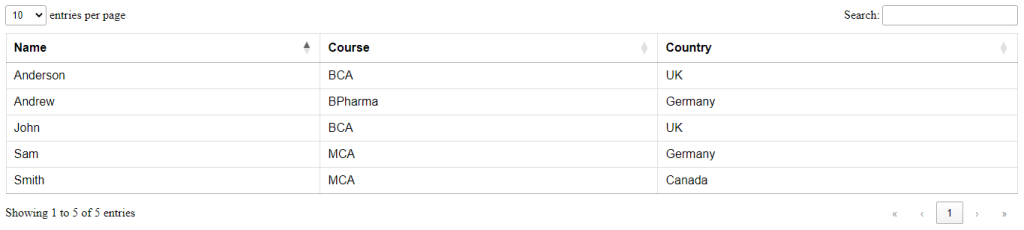
Join 10,000+ subscriber